programmatically create a TabBarController application
We can create application by choose File->New Project ,and select 'window-based application'
Give a name (say tabbarsample) and save the project
create tableviewcontroller file to display when click on tab
File->New File, select UIViewController subclass, and check the UITableViewController subclass
give a name (example MyTableViewController)
create an instance of NSObject file to retrieve data from that class
File->New File, select Objective-C class, and select subclass of NSObject
give file name example DataAccess
After doing these steps, we can see six files in our classes folder
This is the final product :
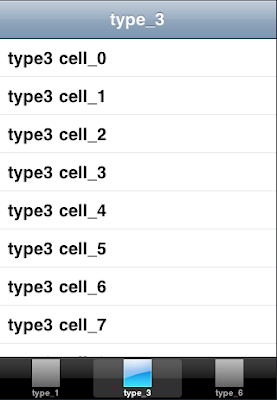
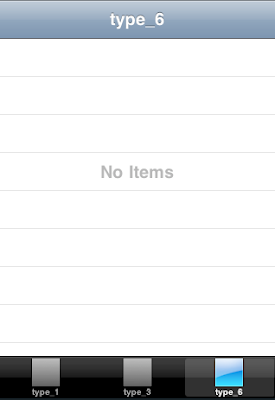
Its better to show the code in all six files, it will tell the remaining part..
used iPhone sdk 3.1
tested with Simulator 3.1/Debug
thanks to reading..:)
We can create application by choose File->New Project ,and select 'window-based application'
Give a name (say tabbarsample) and save the project
create tableviewcontroller file to display when click on tab
File->New File, select UIViewController subclass, and check the UITableViewController subclass
give a name (example MyTableViewController)
create an instance of NSObject file to retrieve data from that class
File->New File, select Objective-C class, and select subclass of NSObject
give file name example DataAccess
After doing these steps, we can see six files in our classes folder
This is the final product :
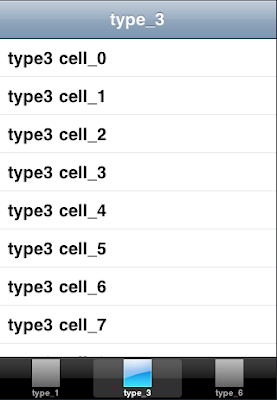
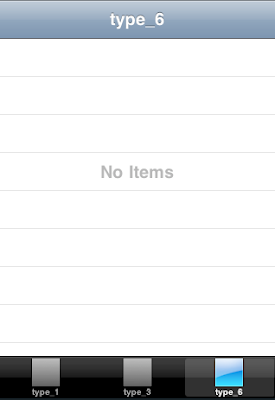
Its better to show the code in all six files, it will tell the remaining part..
//
// tabbarsampleAppDelegate.h
// tabbarsample
#import <UIKit/UIKit.h>
@interface tabbarsampleAppDelegate : NSObject <UIApplicationDelegate, UITabBarControllerDelegate> {
UIWindow *window;
UITabBarController *mytabBarController;
}
@property (nonatomic, retain) IBOutlet UIWindow *window;
@property (nonatomic, retain) UITabBarController *mytabBarController;
@end
//
// tabbarsampleAppDelegate.m
// tabbarsample
//
#import "tabbarsampleAppDelegate.h"
#import "MyTableViewController.h"
@implementation tabbarsampleAppDelegate
@synthesize window;
@synthesize mytabBarController;
- (void)applicationDidFinishLaunching:(UIApplication *)application {
mytabBarController = [[UITabBarController alloc] init];
NSMutableArray *array = [[NSMutableArray alloc] init];
MyTableViewController *myTableViewController1 = [[MyTableViewController alloc] initWithParam:1];
UINavigationController *myTableNavController1 = [[UINavigationController alloc] initWithRootViewController:myTableViewController1] ;
[array addObject:myTableNavController1];
[myTableViewController1 release];
[myTableNavController1 release];
myTableViewController1 = nil;
myTableNavController1 = nil;
MyTableViewController *myTableViewController2 = [[MyTableViewController alloc] initWithParam:3];
UINavigationController *myTableNavController2 = [[UINavigationController alloc] initWithRootViewController:myTableViewController2] ;
[array addObject:myTableNavController2];
[myTableViewController2 release];
[myTableNavController2 release];
myTableViewController2 = nil;
myTableNavController2 = nil;
MyTableViewController *myTableViewController = [[MyTableViewController alloc] initWithParam:6];
UINavigationController *myTableNavController = [[UINavigationController alloc] initWithRootViewController:myTableViewController] ;
[array addObject:myTableNavController];
[myTableViewController release];
[myTableNavController release];
myTableViewController = nil;
myTableNavController = nil;
window.autoresizingMask = UIViewAutoresizingFlexibleWidth | UIViewAutoresizingFlexibleHeight;
mytabBarController.view.autoresizingMask = UIViewAutoresizingFlexibleWidth | UIViewAutoresizingFlexibleHeight;
mytabBarController.viewControllers = array;
[window setBackgroundColor:[UIColor whiteColor]];
[window addSubview:mytabBarController.view];
mytabBarController.delegate = self;
[array release];
// Override point for customization after application launch
[window makeKeyAndVisible];
}
- (void)dealloc {
[mytabBarController release];
[window release];
[super dealloc];
}
#pragma mark UITabBarControllerDelegate
- (void)tabBarController:(UITabBarController *)tabBarController didEndCustomizingViewControllers:(NSArray *)viewControllers changed:(BOOL)changed{
if(changed){
//write the object to persistent stroe to retrive on next launch
}
}
- (void)tabBarController:(UITabBarController *)tabBarController didSelectViewController:(UIViewController *)viewController{
NSLog(@"clicked index at %i",tabBarController.selectedIndex);
}
@end
//
// MyTableViewController.h
// tabbarsample
//
#import
@interface MyTableViewController : UITableViewController {
NSArray *arrayData;
}
@property(nonatomic, retain) NSArray *arrayData;
- (id)initWithParam:(NSUInteger)param;
@end
//
// MyTableViewController.m
// tabbarsample
//
#import "MyTableViewController.h"
#import "DataAccess.h"
@implementation MyTableViewController
@synthesize arrayData;
- (id)initWithParam:(NSUInteger)param {
// Override initWithStyle: if you create the controller programmatically and want to perform customization that is not appropriate for viewDidLoad.
if (self = [super init]) {
DataAccess *dataAccess = [[DataAccess alloc]init];
self.arrayData = [dataAccess getDataForType:param];
[dataAccess release];
self.title = [NSString stringWithFormat:@"type_%i", param];
self.tabBarItem.image = [UIImage imageNamed:@"images.png"];
//self.tabBarItem.tag = param;//can use for CustomizingViewControllers
}
return self;
}
- (void)didReceiveMemoryWarning {
// Releases the view if it doesn't have a superview.
[super didReceiveMemoryWarning];
// Release any cached data, images, etc that aren't in use.
}
- (void)viewDidUnload {
// Release any retained subviews of the main view.
// e.g. self.myOutlet = nil;
}
#pragma mark Table view methods
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView {
return 1;
}
// Customize the number of rows in the table view.
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
NSUInteger countt = [arrayData count];
if(countt>0){
return [arrayData count];
}
else{
return 4;//to show "No Items" on 4rd cell
}
}
// Customize the appearance of table view cells.
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
UITableViewCell *cell = nil;
if([arrayData count] > 0){
static NSString *CellIdentifier = @"Cell";
cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
cell = [[[UITableViewCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:CellIdentifier] autorelease];
}
// Set up the cell...
cell.textLabel.text = [arrayData objectAtIndex:indexPath.row];
}else{
static NSString *CellIdentifier = @"NoData";
cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
cell = [[[UITableViewCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:CellIdentifier] autorelease];
}
cell.selectionStyle = UITableViewCellSelectionStyleNone;
// Set up the cell...
if(indexPath.row == 3){
cell.textLabel.text= @"No Items";
cell.textLabel.textAlignment= UITextAlignmentCenter;
cell.textLabel.textColor= [UIColor lightGrayColor];
}else{
cell.textLabel.text = @"";
}
}
return cell;
}
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath {
}
- (void)dealloc {
[arrayData release];
[super dealloc];
}
@end
//
// DataAccess.h
// tabbarsample
//
#import
@interface DataAccess : NSObject {
}
-(NSArray *)getDataForType:(NSUInteger)typeOfData;
@end
//
// DataAccess.m
// tabbarsample
//
#import "DataAccess.h"
@implementation DataAccess
-(NSArray *)getDataForType:(NSUInteger)typeOfData{
//to test "No Items"
if(typeOfData == 6){
NSMutableArray *array = [[NSMutableArray alloc] init];
return [array autorelease];
}else
if(typeOfData == 1){
NSArray *array = [NSArray arrayWithObjects:@"type1 cell0",@"type1 cell1",@"type1 cell2",@"type1 cell3",nil];
return array;
}else{
NSMutableArray *array = [[NSMutableArray alloc] init];
for(int i=0 ; i<3*typeofdata>
[array addObject:[NSString stringWithFormat:@"type%i cell_%i",typeOfData,i]];
}
return [array autorelease];
}
}
@end
used iPhone sdk 3.1
tested with Simulator 3.1/Debug
thanks to reading..:)
Thanks Buddy really it helps me a lot..
ReplyDeleteIts my pleasure.. Thanks..
ReplyDeleteThank u David
ReplyDelete